Question & Answer
Question
How can I enable encryption and secure data storage in apps that I develop?
Cause
Secure data and passwords appear in clear text.
Answer
Encrypt and secure sensitive data and passwords in apps that you develop by using the QRadar Python encdec module for secure storage and to encrypt and decrypt sensitive information.
Note: The PyCrypto libraries in your app are subject to US export classification laws. If the app is to be used outside of the US, you must ensure that you have an Export Control Classification Number (ECCN) to comply with United States Department of Commerce regulations.
In the following code snippet, the encdec module is used to encrypt and store a password and a key token. Then, the module is used to retrieve and decrypt those encrypted data items.
from encdec import Encryption
app_password_handler = Encryption({
- 'name': 'appPassword',
'user': 238,
'sec_token': '21afc34e-1d7f-4f9a-a55a-2215582e85bf'
app_password_handler.encrypt('clearTextPasswordSuppliedByUser')
key_token_handler = Encryption({
- 'name': 'keyToken',
'user': 238,
'sec_token': '21afc34e-1d7f-4f9a-a55a-2215582e85bf'
key_token_handler.encrypt('773d3efcb4dd211c213ebf4c45cd81ae')
...
retrieved_password = app_password_handler.decrypt()
key_token = key_token_handler.decrypt()
Here's a copy of the code snippet that you can download:
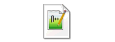
How to use the code
Create a dedicated instance of the encryption object for each data item that you want to manage with the encdec module.
For example, in the previous code snippet, the app_password_handler object manages the password and the key_token_handler object manages the key token for the user who is identified as 238.
The following table describes the elements that are required to create an encryption object.
Encryption object elements | Description |
name | Unique string that identifies the data item to manage. |
user | Unique identifier for the user who owns the data item to manage. |
sec_token | QRadar Authorized Service token with ADMIN capabilities. The sec_token is inserted into the HTTP SEC header, which is used to make REST requests to the QRadar reference_data API. The encdec module uses the QRadar reference_data API to secure data. |
For each user, the encdec module stores the user’s secure data in the /store/<user_ID>_e.db file in the Docker container. For example, secure data for the user identified as 238 in the example code snippet is stored in the container’s /store/238_e.db file.
When you create the encryption object you can use the following functions:
- The encrypt function is invoked to encrypt and securely store a user-data item.
- The decrypt() function is invoked to retrieve and decrypt a user-data item. You can use the same encryption object instance that was used for encryption. If that object is no longer in scope you can instantiate a new encryption object by supplying the same initialization parameters that you used originally.
How to integrate the encdec module into your app
Download the encdec module ( encdec.py) that is attached to this technote and add it to your app's Python codebase. You can compile the encdec.py file with any version of Python.
The encdec module depends on the PyCrypto module, which you can download from the Python website (https://pypi.python.org/pypi/pycrypto). Add the PyCrypto 2.6.1 package and its dependencies to your app's src_deps/pip directory.
encdec.py
The sha256sum for this file is: 66e842f7db1e3fad95cfe3d919990a23
Where do you find more information?

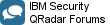



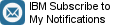

Was this topic helpful?
Document Information
Modified date:
16 June 2018
UID
swg22008898