Using profile-directed feedback
You can use profile-directed feedback (PDF) to tune the performance of your application for a typical usage scenario. The compiler optimizes the application based on an analysis of how often branches are taken and blocks of code are run.
Use PDF process as one of the last steps of optimization before putting the application into production. Optimization at all levels from -O2 up can benefit from PDF. Other optimizations such as the -qipa option and optimization levels -O4 and -O5 can also benefit when using with PDF process.
The following diagram illustrates the PDF process:
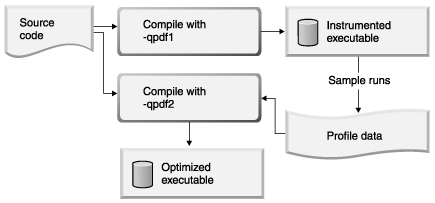
To use the PDF process to optimize your application, follow these steps:
Compile some or all of the source files in a program with the -qpdf1 option. You must specify at least the -O2 optimization level.
Notes:- A PDF map file is generated at this step. It is used for the showpdf utility to display part of the profiling information in text or XML format. For details, see Viewing profiling information with showpdf. If you do not need to view the profiling information, specify the -qnoshowpdf option at this step so that the PDF map file is not generated. For details of -qnoshowpdf, see -qshowpdf.
- Although you can specify PDF optimization (-qpdf) as early in the optimization level as -O2, PDF optimization is recommended at -O4 and higher.
- You do not have to compile all of the files of the programs with the -qpdf1 option. In a large application, you can concentrate on those areas of the code that can benefit most from the optimization.
- When option -O4, -O5, or any level of option -qipa is in effect, and you specify the -qpdf1 option at the link step but not at the compile step, the compiler issues a warning message. The message indicates that you must recompile your program to get all the profiling information.
Restriction: When you run an application that is compiled with -qpdf1, you must end the application using normal methods, including reaching the end of the execution for the main function and calling the exit() function in libc (stdlib.h) for C/C++ programs. System calls exit(), _Exit(), and abort() are considered abnormal termination methods and are not supported. Using abnormal program termination might result in incomplete instrumentation data generated by using the PDF file or PDF data not being generated at all.Run the resulting application with a typical data set. When the application exits, profile information is written to one or more PDF files. You can train the resulting application multiple times with different data sets. The profiling information is accumulated to provide a count of how often branches are taken and blocks of code are run, based on the input data used. This step is called the PDF training step.By default, the PDF file is named ._pdf, and it is placed in the current working directory or the directory specified by the PDFDIR environment variable. If the PDFDIR environment variable is set but the specified directory does not exist, the compiler issues a warning message. To override the defaults, use the -qpdf1=pdfname or -qpdf1=exename option.
If you recompile your program by using either of the -qpdf1=level=0 or -qpdf1=level=1 option, single-pass profiling is supported. The compiler removes the existing PDF file before generating a new application.
If you recompile your program by using -qpdf1=level=2 option, multiple-pass profiling is supported. You can repeat compiling your program and training the resulting application, then new PDF files are generated up to five times.
Notes:- When you compile your program with the -qpdf1 or -qpdf2 option, by default, the -qipa option is also invoked with level=0.
- To avoid wasting compile and run time, make sure that the PDFDIR environment variable is set to an absolute path. Otherwise, you might run the application from a wrong directory, and the compiler cannot locate the profiling information files. When it happens, the program might not be optimized correctly or might be stopped by a segmentation fault. A segmentation fault might also happen if you change the value of the PDFDIR environment variable and run the application before the PDF process finishes.
- Avoid using atypical data that can distort the analysis to infrequently executed code paths.
- If you have several PDF files, use the mergepdf utility to combine these PDF files into one PDF file. For example, if you produce three PDF files that represent usage patterns that occur 53%, 32%, and 15% of the time respectively, you can use this command:
mergepdf -r 53 path1 -r 32 path2 -r 15 path3
Notes:- Avoid mixing the PDF files created by different versions or PTF levels of the XL C/C++ compiler.
- You cannot edit PDF files that are generated by the resulting application. Otherwise, the performance or function of the generated executable application might be affected.
Recompile your program using the same compiler options as before, but change -qpdf1 to -qpdf2. In this second compilation, the accumulated profiling information is used to fine-tune the optimizations. The resulting program contains no profiling overhead and runs at full speed.
Notes:- If the compiler cannot read any PDF files in this step, the compiler issues error message 1586-401 but continues the compilation.
- You are highly recommended to use the same optimization level at all compilation steps for a particular program. Otherwise, the PDF process cannot optimize your program correctly and might even slow it down. All compiler settings that affect optimization must be the same, including any supplied by configuration files.
- You can modify your source code and use the -qpdf1 and -qpdf2 options to compile your program. Old profiling information can still be preserved and used during the second stage of the PDF process. The compiler issues a list of warnings but the compilation does not stop. An information message is also issued with a number in the range of 0 - 100 to indicate how outdated the old profiling information is.
- When option -O4, -O5, or any level of option -qipa is in effect, and you specify the -qpdf2 option at the link step but not at the compile step, the compiler issues a warning message. The message indicates that you must recompile your program to get all the profiling information.
- When using the -qreport option with the -qpdf2 option, you can get additional information in your listing file to help you tune your program. This information is written to the PDF Report section.
If you want to erase the PDF information, use the cleanpdf or resetpdf utility.
Instead of step 4, you can use the -qpdf2 option to link the object files that are created during the -qpdf1 phase without recompiling your program during the -qpdf2 phase. This alternative approach can save considerable time and help tune large applications for optimization.
Examples
#Set the PDFDIR variable
export PDFDIR=$HOME/project_dir
#Compile most of the files with -qpdf1
xlc -qpdf1 -O3 -c file1.c file2.c file3.c
#This file does not need optimization
xlc -c file4.c
#Non-PDF object files such as file4.o can be linked
xlc -qpdf1 -O3 file1.o file2.o file3.o file4.o
#Run several times with different input data
./a.out < polar_orbit.data
./a.out < elliptical_orbit.data
./a.out < geosynchronous_orbit.data
#No need to recompile the source of non-PDF object files
#(file4.c).
xlc -qpdf2 -O3 -c file1.c file2.c file3.c
#Link all the object files into the final application
xlc -qpdf2 -O3 file1.o file2.o file3.o file4.o
#Compile source with -qpdf1
xlc -c -qpdf1 -O3 file1.c file2.c
#Link object files
xlc -qpdf1 -O3 file1.o file2.o
#Run with one set of input data
./a.out < sample.data
#Link object files
xlc -qpdf2 -O3 file1.o file2.o