Service routines
Under Language Environment, you can specify several service routines to execute a main routine or subroutine in the preinitialized environment. To use the routines, specify a list of addresses of the routines in a service routine vector as shown in Figure 1.
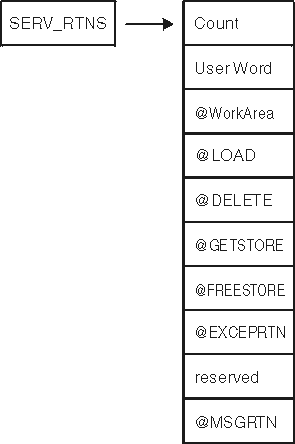
The service routine vector is composed of a list of fullword addresses of routines that are used instead of Language Environment service routines. The list of addresses is preceded by the number of the addresses in the list, as specified in the count field of the vector. The service_rtns parameter that you specify in calls to (init_main) and (init_sub) contains the address of the vector itself. If this pointer is specified as zero (0), Language Environment routines are used instead of the service routines shown in Figure 1.
The @GETSTORE and @FREESTORE service routines must be specified together; if one is zero, the other is automatically ignored. The same is true for the @LOAD and @DELETE service routines. If you specify the @GETSTORE and @FREESTORE service routines, you do not have to specify the @LOAD and @DELETE service routines and vice-versa.
When replacing only the storage management routines without the program management routines, the user must be aware that they may not be accounting for all the storage obtained on behalf of the application. Contents management obtains storage for the load module being loaded. This storage will not be managed by the user storage management routines.
- Count
- A fullword binary number representing the number of fullwords that follow. The count does not include itself. In Figure 1, the count is 9. For each vector slot, a zero represents the absence of the routine, a nonzero represents the presence of a routine.
- User Word
- A fullword that is passed to the service routines. The user word is provided as a means for your routine to communicate to the service routines.
- @WorkArea
- An address of a work area of at least 256 bytes that is doubleword aligned. The first word of the area contains the length of the area provided. This parameter is required if service routines are present in the service routine vector. This length field must be initialized each time you bring up a new PreInit environment.
- @LOAD
- This routine loads named routines for application management.
The parameter that is passed contains the following:
- Name_addr
- The fullword address of the name of the module to load (input parameter).
- Name_length
- A fixed binary(31) length of the module name (input parameter).
- User_word
- A fullword user field (input parameter).
- Load_point
- Either zero (0), or the address where the @LOAD routine is to store the load point address of the loaded routine (input and output parameter).
- Entry_point
- The fullword entry point address of the loaded routine (output parameter).
- Module_size
- The fixed binary(31) size of the module that was loaded (output parameter).
- Return code
- The fullword return code from load (output).
- Reason code
- The fullword reason code from load (output). The return and reason
codes are listed in Table 1.
Table 1. Return and reason codes from load (output) Return code Reason code Description 0 0 Successful 0 12 Successful; loaded using SVC8 4 4 Unsuccessful; module loaded above the line when in AMODE(24) 8 4 Unsuccessful; load failed 16 4 Unsuccessful; uncorrectable error occurred
- @DELETE
- This routine deletes routines for application management. The
parameter that is passed contains the following:
- Name_addr
- The fullword address of the module name to be deleted (input parameter).
- Name_length
- A fixed binary(31) length of module name (input parameter).
- User_word
- A fullword user field (input parameter).
- Rsvd_word
- A fullword reserved for future use (input parameter); must be zero.
- Return code
- The return code from delete service (output).
- Reason code
- The reason code from delete service (output). The return and reason
codes are listed in Table 2.
Table 2. Return and reason codes from delete service (output) Return code Reason code Description 0 0 Successful 8 4 Unsuccessful; delete failed 16 4 Unsuccessful; uncorrectable error occurred
- @GETSTORE
- This routine allocates storage on behalf of the storage manager.
This routine can rely on the caller to provide a save area, which
can be the @Workarea. The parameter list that is passed contains the following:
- Amount
- A fixed binary(31) amount of storage requested (input parameter).
- Subpool_no
- A fixed binary(31) subpool number 0-127 (input parameter). Language Environment allocates storage from the process-level storage pools.
- User word
- A fullword user field (input parameter).
- Flags
- A fullword flag area (input parameter), as shown in the following
table. The remaining flag bits are reserved for future use and must
be zero.
Bit Setting Description Zero ON The storage required must be allocated below the 16 MB line. OFF The storage required can be allocated anywhere. One ON The storage required was requested to be backed by 1 MB pages. This setting might be ignored. OFF The storage required was requested to be backed by the default 4 KB pages. - Stg_address
- The fullword address of the storage obtained or zero (output parameter).
- Obtained
- A fixed binary(31) number of bytes obtained (output parameter).
- Return code
- The return code from @GETSTORE service (output parameter).
- Reason code
- The reason code from the @GETSTORE service (output parameter).
The return and reason codes are listed in Table 3.Table 3. Return and reason codes from the @GETSTORE service Return code Reason code Description 0 0 Successful 16 0 Unsuccessful; uncorrectable error occurred - @FREESTORE
- This routine frees storage on behalf of the storage manager. The
parameter list passed contains the following:
- Amount
- The fixed binary(31) amount of storage to free (input parameter).
- Subpool_no
- The fixed binary(31) subpool number 0-127 (input parameter). Language Environment allocates storage from the process-level storage pools.
- User word
- A fullword user field (input parameter).
- Stg_address
- The fullword address of the storage to free (input parameter).
- Return code
- The return code from the @FREESTORE service (output).
- Reason code
- The reason code from the @FREESTORE service (output).
The return and reason codes are listed in Table 4.Table 4. Return and reason codes from the @FREESTORE service Return code Reason code Description 0 0 Successful 16 0 Unsuccessful; uncorrectable error occurred - @EXCEPRTN
- This routine traps program interruptions and abends for condition
management. The parameter list passed contains the following:
- Handler_addr
- During an initialization call, this parameter contains the address of the Language Environment® condition handler. During a termination call, this parameter contains a pointer to a fullword field containing zeroes.
- Environment_token
- A fullword Recovery Environment token (input). This token is different from the Preinitialization environment token used with CEEPIPI calls.
- User_word
- A fullword user field (input parameter)
- Abend_flags
- A fullword flag area containing abend flags (input)
- Check_flags
- A fullword flag area containing program check flags (input)
- Return code
- The return code from the @EXCEPRTN service (output).
- Reason code
- The reason code from the @EXCEPRTN service (output).
The exception router is responsible for trapping and routing exceptions. These are the services typically obtained via the ESTAE and ESPIE macros.
During initialization, Language Environment puts the address of the Language Environment condition handler in the first field of the above parameter list, and sets the environment token field to a value that must be passed on to the Language Environment condition handler. It also sets abend and check flags as appropriate, and then calls your exception router to establish an exception handler.
The meaning of the bits in the abend flags are given by the following declare:dcl 1 abendflags, 2 system, 3 abends bit(1), /* control for system abends desired */ 3 rsrv1 bit(15), /* reserved */ 2 user, 3 abends bit(1), /* control for user abends desired */ 3 rsrv2 bit(15); /* reserved */
The meaning of the bits in the check flags is given by the following declare:1 checkflags, 2 type, 3 reserved3 bit(1), 3 operation bit(1), 3 privileged_operation bit(1), 3 execute bit(1), 3 protection bit(1), 3 addressing bit(1), 3 specification bit(1), 3 data bit(1), 3 fixed_overflow bit(1), 3 fixed_divide bit(1), 3 decimal_overflow bit(1), 3 decimal_divide bit(1), 3 exponent_overflow bit(1), 3 exponent_underflow bit(1), 3 significance bit(1), 3 float_divide bit(1), 2 reserved4 bit(16);
The return and reason codes that the exception router must use are listed in Table 5.Table 5. Return and reason codes for the exception router Return code Reason code Description 0 0 Successful 4 4 Unsuccessful; the exit could not be established or removed 16 4 Unsuccessful; unrecoverable error occurred When an exception occurs, the exception handler must determine if the Language Environment condition handler is interested in the exception (by examining abend and check flags). If the condition handler is not interested in the exception, the exception handler must treat the program as in error, but can assume the environment for the thread to be functional and reusable. If the condition handler is interested in the exception, the exception handler must invoke the condition handler, passing the parameters listed in Table 6.Table 6. Parameters for Language Environment condition handler Parameter Attributes Type Environment Token Fixed Bin(31) Input Address of SDWA Pointer Input Return Code Fixed Bin(31) Output Reason Code Fixed Bin(31) Output The return and reason codes upon return from the Language Environment condition handler are listed in Table 7.Table 7. Return and reason codes from the Language Environment condition handler Return code Reason code Description 0 0 Continue with the exception. Percolate the exception taking whatever action would have been taken had it not been handled at all. In this case, your exception handler can assume the environment for the thread to be functional and reusable.
0 4 Continue with the exception. Percolate the exception taking whatever action would have been taken had it not been handled at all. In this case, the environment for the thread is probably unreliable and not reusable. A forced termination is suggested.
4 0 Resume execution using the updated SDWA. The invoked Language Environment condition handler will have already used the SETRP RTM macro to set the SDWA for correct resumption.
During termination, the exception router is invoked with the condition handler address (first parameter) set to zero to de-establish the exit (if it was established during initialization).
When a nested enclave is created, Language Environment calls the exception router to establish another exception handler exit, and then makes a call to de-establish it when the nested enclave terminates. If an exception occurs while the second exit is active, special processing is performed. Depending on what this second exception is, either the first exception will not be retried, or processing will continue on the first exception by requesting retry for the second exception.
If the Language Environment condition handler determines that execution should resume for an exception, it will set the SDWA with SETRP and return with return/reason codes 4/0. Execution will resume in library code or in user code, depending on what the exception was.
The exception handler must be capable of restoring all the registers from the SDWA when control is given to the retry routine. The ESPIE and ESTAE services are capable of accomplishing this.
In using the exception router service:- The exception handler should not invoke the Language Environment condition handler if active I/O has been halted and is not restorable.
- This service requires an XA or ESA environment.
If an exception occurs while the exception handler is in control before another exception handler exit has been stacked, the exception handler should assume that the exception could not be handled and that the environment for the program (thread) is damaged. In this case, the exception handler should force termination of the preinitialized environment.
When @EXCEPRTN is specified, the following items are not supported:- XPLINK applications
- POSIX(ON) applications
- DYNDUMP settings other than DYNDUMP(,NODYNAMIC)
- IMS™ applications
- Applications that use Binary Floating Point (BFP) or Decimal Floating Point (DFP) numbers
- Applications that use the Compare-and-Trap family of instructions
Notes:- If the passed-in SDWA from the exception handler to the Language Environment condition handler does not contain valid high registers, the "HR_VALID" flag bit in the Machine State "FLAGS" field will be off, indicating that the saved high registers are not valid.
- If a nested enclave ends because of an unhandled condition and a 4094-40 ABEND is declared, the high registers may not be valid in the Machine State that contains information about the 4094-40 ABEND.
- If registers in the passed-in SDWA at the time of interrupt (in the SDWAGRSV field) are not appropriate or recognizable, and Language Environment instead saves the registers from the SDWASRSV field in the Machine State, the high registers may not be valid in the Machine State.
- @MSGRTN
- This routine allows error messages to be processed by the caller
of the application. If the message pointer is zero, your message routine is expected to return the size of the line to which messages are written (in the line_length field). This allows messages to be formatted correctly; that is, messages can be broken at places such as blanks.
- Message
- A pointer to the first byte of text that is printed, or zero (input parameter).
- Msg_len
- The fixed binary(31) length of the message (input parameter).
- User word
- A fullword user field (input parameter).
- Line_length
- The fixed binary(31) size of the output line length. This is used when Message is zero (output parameter).
- Return and reason codes
- Two fullwords containing the return and reason codes listed in Table 8 (output parameters).
Table 8. Return and reason codes for the @MSGRTN service Return code Reason code Description 0 0 Successful 16 4 Unsuccessful; uncorrectable error occurred